Click Without Triggering: Engineering a Zero-Interference Element Selector
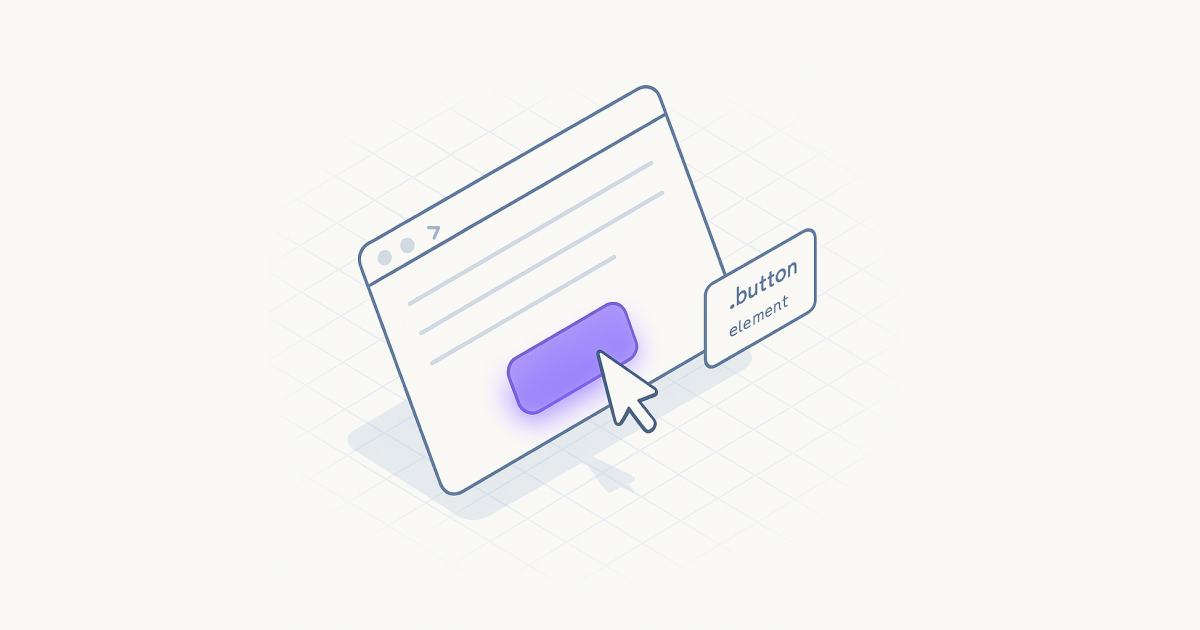
Yesterday I had a chat with one of BrowserUse's co-founders, Magnus Müller, and as I was showing him how our Element Selector extension he had questions around how we prevent default actions on anchors and buttons during element selection. After the call, I thought this would make a good Engineering blog post.
So today, I'll share how we built our element inspector that allows users to select and analyze DOM elements without triggering their default behaviors.
The Challenge
When building a DOM element inspector, one of the primary challenges is handling interactive elements like links and buttons. The goal is to:
Allow users to inspect these elements
Prevent navigation or form submissions
Maintain visual feedback
Keep the original page structure intact
Solution Architecture
We'll explore three different approaches, each with its own use cases and trade-offs.
1. The Event Prevention Approach
This is the foundational layer of our solution.
Let's break down our base ElementInspector class - the foundation of our non-intrusive DOM selection tool.
The constructor sets up two critical properties:
isActive: A boolean flag that tracks whether the inspector is currently enabled
boundPreventDefault: A properly bound event handler that maintains the correct this context Binding the event handler in the constructor is an important performance optimization. Without it, we'd need to create a new function binding every time we attach an event listener, which would be inefficient and potentially create memory leaks.
This method is our event handler, deployed as a strategic interceptor for user interactions. It performs three critical actions:
event.preventDefault(): Cancels the default behavior of the element (like navigation for links or form submission for buttons)
event.stopPropagation(): Prevents the event from bubbling up through the DOM, ensuring no parent elements receive the event
this.handleElementSelection(event.target): Invokes our element selection logic with the target DOM node
The if (this.isActive) check provides a failsafe mechanism - even if our event listeners are somehow still attached, they won't take action unless the inspector is active.
The enable() method activates our inspector by:
Setting isActive to true
Attaching our event handler to multiple event types
Using the event capturing phase (true as the third parameter)
The event capturing phase is critical here. Most JavaScript events go through three phases:
Capturing phase (down the DOM tree)
Target phase (at the target element)
Bubbling phase (back up the DOM tree)
By using the capturing phase, we intercept events before they reach their target elements, allowing us to prevent default behaviors before they're triggered.
We listen for multiple event types to ensure comprehensive coverage:
click: Captures most interactive element behaviors
mousedown/mouseup: Catches drag-based interactions and some custom widgets
submit: Specifically targets form submissions that might not trigger click events
2. The Overlay Approach
This is our primary solution at Samelogic, offering the best balance of performance and user experience.
3. The Iframe Approach (For Complex Cases)
For handling edge cases and complex single-page applications:
Best Practices and Lessons Learned
1. Performance Optimization
To ensure smooth performance, especially during mousemove events:
2. Accessibility Considerations
Make sure your inspector is keyboard-accessible:
3. Memory Management
Proper cleanup is crucial for extensions:
Integration Example
Here's how to put it all together:
In closing, building a robust element inspector requires careful consideration of various factors:
Event handling and prevention
Visual feedback
Performance optimization
Accessibility
Memory management
At Samelogic, we've found that the combination of event prevention and overlay approach provides the best user experience while maintaining performance. The iframe approach serves as a reliable fallback for complex cases.Remember to:
Always provide visual feedback for user actions
Implement proper cleanup mechanisms
Consider accessibility from the start
Optimize for performance, especially for mousemove events
Handle edge cases gracefully
Try this in our Chrome Extension yourself: 👉 Install our Element Inspector
Understand customer intent in minutes, not months
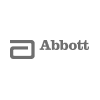
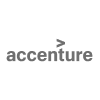
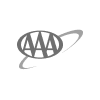
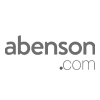
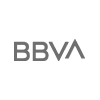
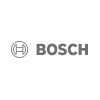
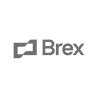
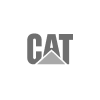
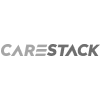
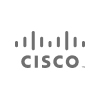
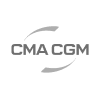
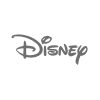
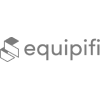
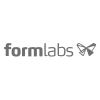
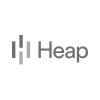
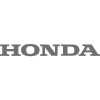
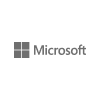
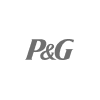
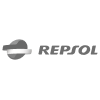
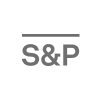
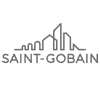
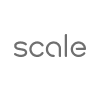
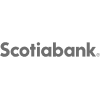
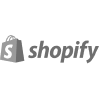
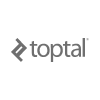
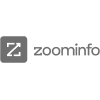
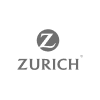
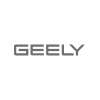
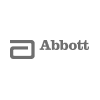
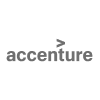
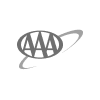
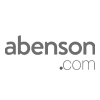
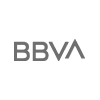
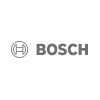
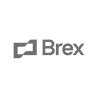
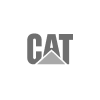
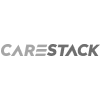
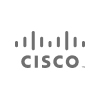
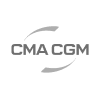
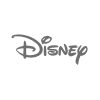
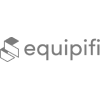
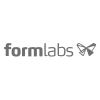
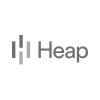
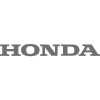
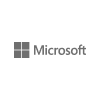
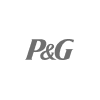
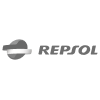
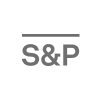
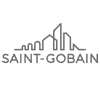
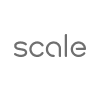
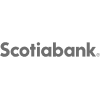
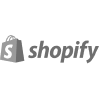
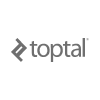
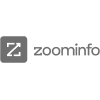
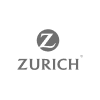
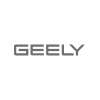