Heap Analytics in NextJs with NextAuthJs
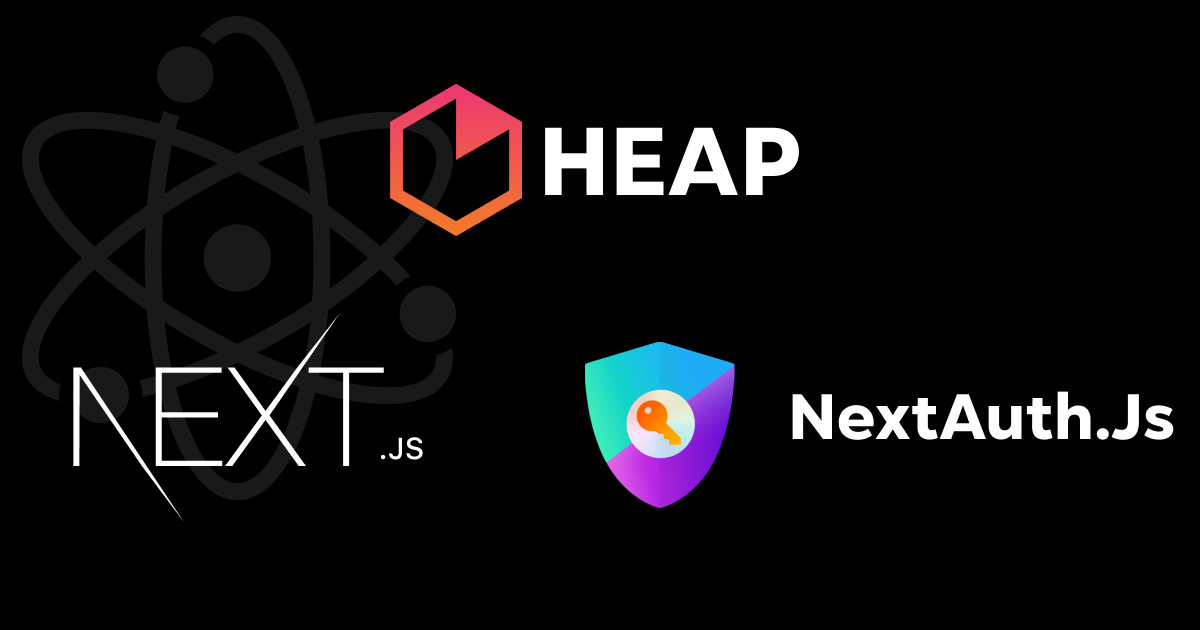
How to set up Heap Analytics Web SDK in NextJs and identify the user with NextAuth.Js.
This way, we can know who is doing what and understand our user behaviors better.
Prerequisites
Heap Analytics Account - Follow the instructions to get the script.
Setup basic authentication with NextAuth.Js - Follow their github instructions.
Methods
Since most web apps use multiple analytics sources, we will structure our code to wrap all analytics under one component.
Our file structure will look like this:
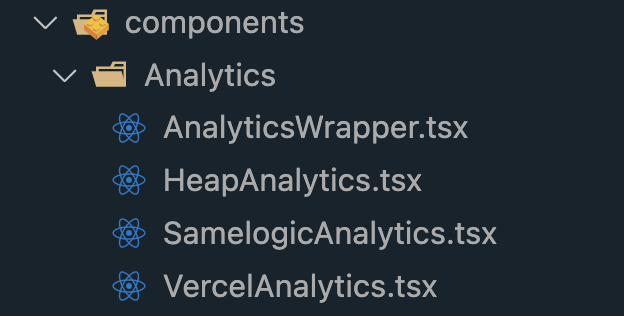
We will reference `AnalyticsWrapper` inside our `_app.tsx` component.
🧾 Let's write code
Lets jump right into the main code.
Here we have an `HeapAnalytics` component that does a few things:
It inject's the heap sdk using the NextJs `Script` component.
When the script is ready, it will signal to `useEffect` to setup the identifier. We use useEffect here to watch the state of `session` and `status` since those will change depending on when the component fetches the data.
We then check the `window.heap` object to ensure heap attached itself to the object and then we make the calls to their API.
We are identifying users by email address, so we take that from the session and assign it to heap.
❗️Note: Set up your environment variables to differentiate between dev and production, or you will contaminate your data.
The `AnalyticsWrapper` is just a wrapper component around the other analytics scripts (even google tag manager).
In your `_app.tsx`, make sure to setup your `<SessionProvider>` first and then place the `AnalyticsWrapper` inside:
Conclusion
We integrated heap to attach identification to the user who logged in on this website using the code above.
A user in heap will look like this:

This seems to be all that is needed for now.
GLHF!
Understand customer intent in minutes, not months
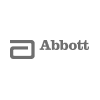
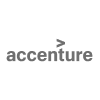
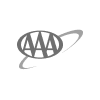
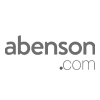
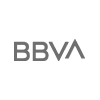
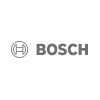
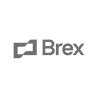
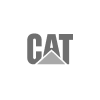
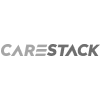
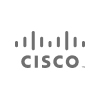
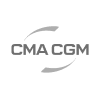
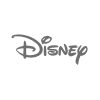
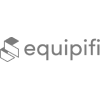
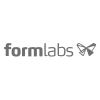
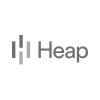
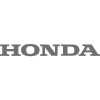
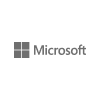
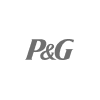
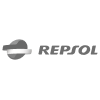
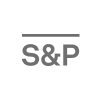
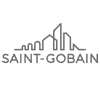
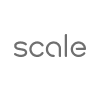
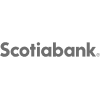
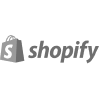
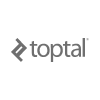
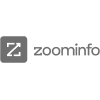
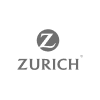
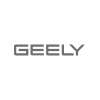
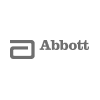
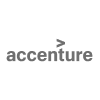
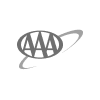
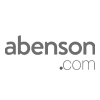
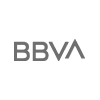
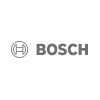
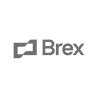
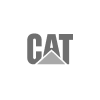
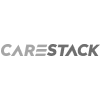
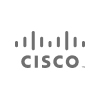
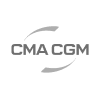
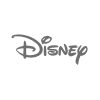
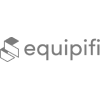
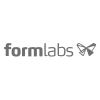
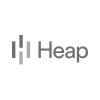
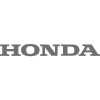
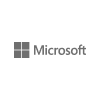
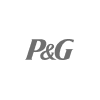
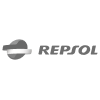
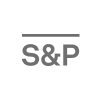
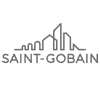
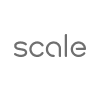
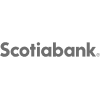
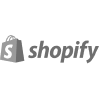
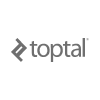
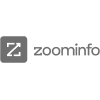
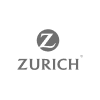
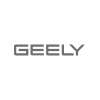